How-to guides
How-to guides
Monitor AI workflow in production
How to find failures in production in your RAG agentic workflows
Get your Maihem API key and install the SDK before you start.
1
Add target agent
In Python, run the following code to add a new target agent.
Python
Copy
from maihem import Maihem
maihem_client = Maihem()
target_agent = maihem_client.add_target_agent(
api_key="<YOUR_API_KEY>", # Optional, pass here if not an environment variable
name="agent_1",
role="Financial advisor",
description="Financial advisor that can help with questions about stocks, bonds, and other financial products",
)
2
Add a decorator to each step of your workflow
This is an example of a basic agentic workflow (that returns hardcoded data). Each step of the workflow is monitored and evaluated by setting a Maihem decorator with its corresponding evaluator.
Copy the code below into a new Python file and call it workflow.py
.
See a full list of supported evaluators and metrics and their required input and output maps.
workflow.py
Copy
import asyncio
import random
import maihem
from maihem.evaluators import (
MaihemIntent,
MaihemNER,
MaihemQueryRewriting,
MaihemSearch,
MaihemReranking,
MaihemFiltering,
MaihemFinalAnswer,
MaihemConversational,
)
from maihem import Maihem, MaihemClient
@maihem.workflow_step(
name="intent_recognition_custom_name",
evaluator=MaihemIntent(
inputs=MaihemIntent.Inputs(query="user_input"),
output_fn=lambda x: x["intent"], # Extract intent from the returned dict
),
)
async def intent_recognition_function(
user_input: str, # Different parameter name than the standard "query"
) -> dict:
# Return richer data
return {
"intent": "Financial Report",
"confidence": 0.95,
"alternatives": ["FAQ", "General Query"],
}
@maihem.workflow_step(evaluator=MaihemNER(inputs=MaihemNER.Inputs(query="text")))
async def name_entity_recognition_function(
text: str,
) -> list[str]: # Different parameter name
return ["Pepsi", "2022"]
@maihem.workflow_step(
evaluator=MaihemQueryRewriting(
inputs=MaihemQueryRewriting.Inputs(query="input_text")
)
)
async def rephrase_query_function(input_text: str) -> str: # Different parameter name
return "What was PepsiCo's financial situation in 2022?"
DOCUMENTS = [
"PepsiCo annual revenue for 2023 was $91.471B, a 5.88% increase from 2022. PepsiCo annual revenue for 2022 was $86.392B, a 8.7% increase from 2021. PepsiCo annual revenue for 2021 was $79.474B, a 12.93% increase from 2020.",
"On a reported basis, the division operating profit percentages were: Frito-Lay North America 45%, Quaker Foods North America 4%, PepsiCo Beverages North America 40%, Latin America 12%, Europe (10%), Africa, Middle East and South Asia 5% and Asia Pacific, Australia and New Zealand and China Region 4% 2021 and 2022 reported operating profit was $11,162 and $11,512, respectively, reflecting an increase of 3% in 2022. ",
"| Metric | 2022 | 2021 | % Change |\n|---------|-------|------|----------|\n| Net revenue | $86,392 | $79,474 | 9% |\n| Core operating profit | $12,325 | $11,414 | 8% |\n| Reported earnings per share | $6.42 | $5.49 | 17% |\n| Core earnings per share | $6.79 | $6.26 | 9% |\n| Free cash flow | $5,855 | $7,157 | -18% |\n| Capital spending | $5,207 | $4,625 | 13% |\n| Common share repurchases | $1,500 | $106 | 1,320% |\n| Dividends paid | $6,172 | $5,815 | 6% |",
"PepsiCo has long been a leader in the global beverage and snack food industry, with a diverse portfolio that includes iconic brands like Pepsi, Lay’s, Gatorade, and Tropicana. In recent years, the company has focused on expanding its healthier product lines, responding to shifting consumer preferences toward low-sugar and organic options. This strategic pivot has involved significant marketing campaigns and product innovation, positioning PepsiCo as a competitor to other giants like Coca-Cola in the evolving market landscape.",
"In 2023, PepsiCo announced a major sustainability initiative aimed at reducing its carbon footprint by 40% by 2030. This plan includes investments in renewable energy for its manufacturing plants and a shift toward recyclable packaging across its product lines. While these efforts reflect the company’s broader corporate responsibility goals, they also signal to investors a long-term commitment to adapting to environmental regulations and consumer expectations, which could influence operational costs and brand perception in the coming years.",
]
@maihem.workflow_step(
evaluator=MaihemSearch(
inputs=MaihemSearch.Inputs(query="search_query"),
output_fn=lambda x: x["documents"], # Extract just the documents
)
)
async def retrieval_function(search_query: str) -> dict: # Different parameter names
random.shuffle(DOCUMENTS)
return {
"documents": DOCUMENTS,
"scores": [0.95, 0.85, 0.75, 0.65, 0.55],
"metadata": [
{"source": "wiki", "date": "2024"},
{"source": "web", "date": "2023"},
{"source": "news", "date": "2024"},
{"source": "book", "date": "2022"},
{"source": "archive", "date": "2021"},
],
}
@maihem.workflow_step(
evaluator=MaihemReranking(
inputs=MaihemReranking.Inputs(query="query", documents="docs")
)
)
async def reranking_function(
query: str, docs: list[str], other_param: str = "default"
) -> list[str]: # Different parameter name
return DOCUMENTS
@maihem.workflow_step(
evaluator=MaihemFiltering(
inputs=MaihemFiltering.Inputs(query="query", documents="documents")
)
)
async def filtering_function(
query: str, documents: list[str]
) -> list[str]: # Different parameter name
return DOCUMENTS[:3]
@maihem.workflow_step(
evaluator=MaihemFinalAnswer(
inputs=MaihemFinalAnswer.Inputs(query="question", documents="context")
)
)
async def answer_function(
question: str, context: list[str]
) -> str: # Different parameter names
return "In 2022, PepsiCo reported strong financial performance, with net revenue of $86.392 billion, an 8.7% increase from $79.474 billion in 2021. Operating profit rose by 3% to $11.512 billion, and earnings per share increased by 17% to $6.42. However, free cash flow decreased by 18% to $5.855 billion, and capital spending increased by 13% to $5.207 billion. Dividends paid to shareholders rose by 6% to $6.172 billion."
@maihem.workflow_step(
name="generate_message",
evaluator=MaihemConversational(
inputs=MaihemConversational.Inputs(query="user_input"),
),
)
async def generate_message(user_input: str) -> str:
####### NOTE THIS
maihem.set_attribute("external_conversation_id", "convconvconv")
###################
intent = await intent_recognition_function(user_input=user_input)
entities = await name_entity_recognition_function(text=user_input)
rephrased_query = await rephrase_query_function(input_text=user_input)
retrieval_results = await retrieval_function(search_query=rephrased_query)
reranking_results = await reranking_function(
query=rephrased_query, docs=retrieval_results["documents"]
)
filtered_results = await filtering_function(
query=rephrased_query, documents=reranking_results
)
result = await answer_function(question=rephrased_query, context=filtered_results)
return result
if __name__ == "__main__":
maihem_client = Maihem(
api_key="<YOUR_API_KEY>",
target_agent_name="agent_1",
env="DEVELOPMENT",
revision="test",
)
asyncio.run(generate_message("What was the Pepsi financial situation in 2022?"))
3
Run your workflow to evaluate it
In the terminal, go to the directory where you created your workflow.py
file, and run it.
Command line interface (CLI)
Copy
python workflow.py
4
Monitor workflow in Maihem UI
Go to your Maihem account, where you can analyze failures that occurred in your workflow in production.
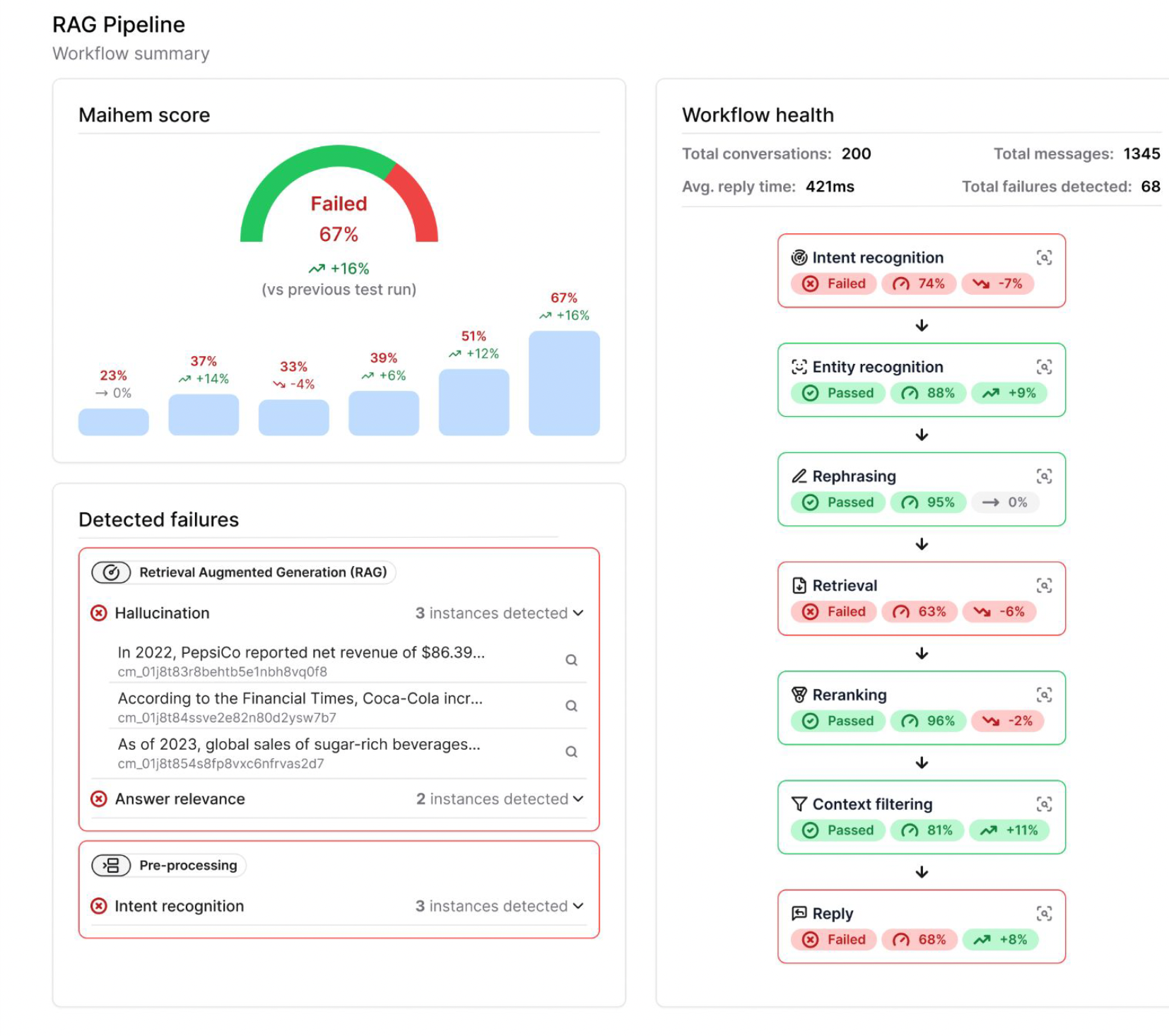
Assistant
Responses are generated using AI and may contain mistakes.